Enter Data With a Visual Studio Office App
In This Topic
This walkthrough shows how to create a Microsoft Visual Studio Office solution that can be used to open InfoConnect and enter data from Excel into a host application. You can use this process to develop an Excel application level add-in that includes these features for any Excel workbook or to customize an Excel workbook so that they are available only when that workbook is open.
Create an Excel workbook add-in
- Create a Microsoft Project and select the Office Add-ins Excel Workbook template or the Excel Add-in template.
- When prompted, select to Create a New Document.
- In Visual Studio, create a new Console Application project and add references for the following InfoConnect assemblies. (Depending on your version of Visual Studio, these can be found either on the .NET tab or under Assemblies | Extensions.)
Attachmate.Reflection
Attachmate.Reflection.Framework
Attachmate.Reflection.Emulation.IbmHosts
Add the Controls to the Workbook's Ribbon
Next, add the controls you want users to see in the Excel Ribbon when they open the document.
- In Solution Explorer, right click on the project and select Add a new item.
- In the Add new item dialog box, select to add a Ribbon (Visual Designer) item to the project.
The Ribbon1.cs file should open in Design mode.
- Open the Toolbox and add a Tab control to the ribbon.
- In the Properties window, change the Tab label to Internal Applications.
- Add a Group control and change its label to Sales Data.
- Add a Button control to this group and change its label to Enter Data.
When you're done, the ribbon shown in design view should look like this:
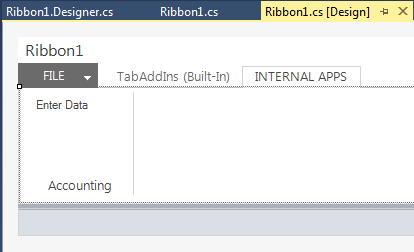
Add the Code for the Controls to the Ribbon1.cs file
Now that you have designed the Ribbon for the workbook, set up the Ribbon.cs file to handle the button click event for the Enter Data button. This code does the work of opening InfoConnect, navigating to the data, and copying the data from Excel to the host application.
You can follow along with this section or skip to Ribbon1.cs code at the end of this article and copy all of the code to Ribbon1.cs.
- Double click the Enter Data button and replace the code in the Ribbon1.cs file with the following code. This code starts InfoConnect and navigates to a screen that has a data form.Then it calls methods to get the data from the Excel worksheet and enter it into the form.
- Add the GetData method to the Ribbon1 partial class. This method gets an Excel range based on the UsedRange property. Then it gets the data in the range row by row and adds it to an array until it gets a delimiter that indicates the end of the data. It returns the array of data.
- Finally, add the EnterData method to the Ribbon.cs partial class. This method enters the data from the spreadsheet into the host, row by row, until all of the data is entered.
Testing the Add-in
- Press F5 to compile and run the sample.
- When the Excel workbook opens, enter a few rows of data in the first four columns of the worksheet.

- Open the INTERNAL APPS tab and then click the Get Data button.
- Verify that InfoConnect opens and navigates to the screen with the data entry form and that the data is entered into the form.