Get Data With a Visual Studio Office App
In This Topic
This walkthrough shows how to create a Microsoft Visual Studio Office solution that can be used to open InfoConnect and get data from a host application. You can use this process to develop an Excel application level add-in that includes these features for any Excel workbook or to customize an Excel workbook so that they are available only when that workbook is open.
Create an Excel workbook add-in
- Create a Microsoft Project and select the Office Add-ins Excel Workbook template or the Excel Add-in template.
- When prompted, select to Create a New Document.
- In Visual Studio, create a new Console Application project and add references for the following InfoConnect assemblies. (Depending on your version of Visual Studio, these can be found either on the .NET tab or under Assemblies | Extensions.)
Attachmate.Reflection
Attachmate.Reflection.Framework
Attachmate.Reflection.Emulation.IbmHosts (if using an IBM host)
Attachmate.Reflection.Emulation.OpenSystems (if using an Open Systems host)
Add Controls to the Excel Ribbon
Next, add the controls you want users to see in the Excel Ribbon when they open the document.
- In Solution Explorer, right click on the project and select Add a new item.
- In the Add new item dialog box, select to add a Ribbon (Visual Designer) item to the project.
The Ribbon1.cs file should open in Design mode.
- Open the Toolbox and add a Tab control to the Ribbon.
- In the Properties window, change the tab label to INTERNAL APPS.
- Add a Group and change its label to Sales Data.
- Add a Button to this group and change its label to Get Data.
When you're done, the Ribbon shown in design view should look like this:
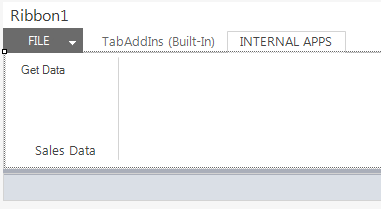
Add the Code for the Controls to the Ribbon1.cs file
Now that you have designed the Ribbon for the workbook, set up the Ribbon1.cs file to handle the button click event for the Get Data button. This code does the work of opening InfoConnect, navigating to the data, and copying the data from the host to Excel.
You can follow along with this section or skip to Ribbon1.cs code at the end of this article and copy all of the code to Ribbon1.cs.
- Double click the Get Data button and replace the code in the Ribbon1.cs file with the following code.
- Add the GetScreenData method to the Ribbon1 partial class. This method gets screen data row by row until it gets a delimiter, indicating the end of the data. Using the resizable List containers allows us to gather any amount of data and resize the containers as we add each row. After the lists are populated, we convert them to a two dimensional array so we can use Excel's method for adding arrays to a worksheet.
- Finally, add the PutDataIntoExcel method to the Ribbon.cs partial class. This method enters the data from the host into a spreadsheet. It creates a range, based on the size of the array that contains the data, and then assigns the array to the range.
Test the Project
- If you are using an Open Systems terminal, create a new VT session using a host name of "demo:UNIX" and save it in the default location (...Documents\Attachmate\InfoConnect) as demoSession.rdox.
- Press F5 to compile and run the sample.
- When the Excel workbook opens, open the INTERNAL APPS tab and then click the Get Data button.
- Verify that InfoConnect opens and navigates to the screen with the data and that the data is entered in the spreadsheet.
The full sample for the Ribbon1.cs file