Dynamically Change the User Interface
In This Topic
You can dynamically show or hide user interface controls or change the actions associated with the controls.
If you prefer to run macros in pre-configured sessions instead of creating your own sessions, you can download the VBA Sample Sessions and open the dynamically-change-the-ui.rd3x (IBM) and dynamically-change-the-ui.rdox (Open Systems) files. The download package contains everything you need to run the macros in these files. See Download the VBA Sample Sessions.
You can show or hide controls based on user credentials or display them only for certain screens.
Display Controls Only for Certain Users
Lets' take a look at how to hide the Tools tab on the Reflection ribbon from users and display it only for administrators.
Note: You can manipulate any control that is configured with the UI Designer. Reflection does not provide programmatic access to other controls.
To create a macro that displays controls for administrators
Before you open Visual Basic, you'll need to get the identifier for the control you want to access in the Reflection UI Designer.
- Open a session in the Reflection workspace and then, on the Appearance tab, select UI Designer.
- In the Reflection UI Designer window, select the control you want to work with (for our example, we'll select the Tools tab).
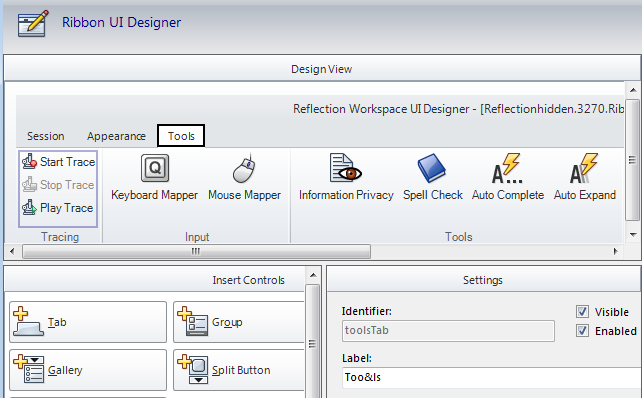
- Note the identifier in the Settings pane. We'll use the "toolsTab" identifier to get access to this control in our macro.
- On the Session tab, select Visual Basic to open the Visual Basic Editor.
- Enter the following code into the ThisIbmTerminal or ThisTerminal (for Open Systems) code window.
Show or Hide Controls on Specific Screens
You can dynamically show or hide user interface controls as screens change.
In this example, we'll create a Reflection demo session that displays a button that runs a macro only when a specific screen is recognized and hides the button on other screens.
To create a macro that shows or hides controls
- Open Reflection and create a demo session as follows:
- For an Ibm session, select Ibm3270 terminal and enter "demo:ibm3270.sim " in the Host name /IP address field.
- For an Open Systems session, select Vt terminal and enter "demo:UNIX" in the Host name /IP address field.
- Open the Visual Basic Editor, insert a code module, and copy the following code into the module.
- On the Appearance tab, select UI Designer.
- In the UI Designer window, select the Session tab and then select the Macros group.
- Under Insert Controls, select Button.
- In the Settings pane, click Select Action to open the Select Action dialog box.
- In the Action list, select Run Reflection Workspace Macro, and then choose the Select Macro option.
- Select the CopyData macro.
- Back in the UI Designer Settings pane, unselect Visible.
- Note the Identifier for the button ("button2") displayed in the Settings window.
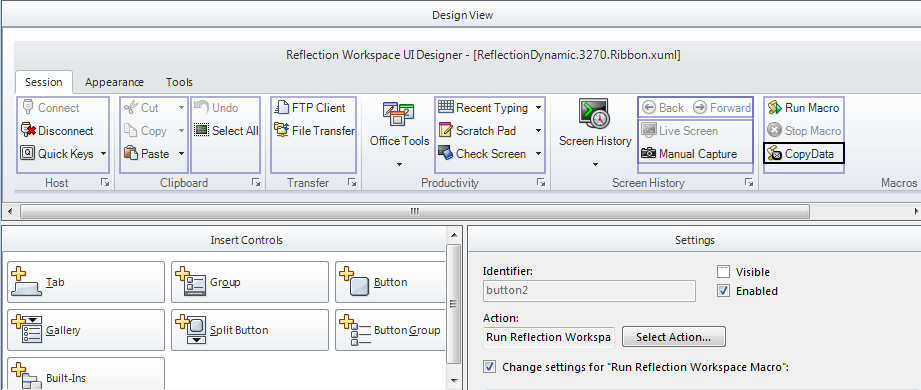
- Close the UI Designer and when you are prompted, save the custom UI file.
Note: The active session is automatically configured to use this custom UI file but you'll need to configure any other sessions to use this file instead of the default built-in. You'll also need to deploy the custom file to your users.
- Back in the Visual Basic editor, enter the following code into the ThisIbmScreen or ThisScreen (for Open Systems) code window.
- Save the session and close it.
Concepts
These samples use events to control macro execution. The first sample handles the BeforeConnect (IBM) and Connecting (Open Systems) events to set the visibility of the control when the session connects. The second sample handles the NewScreenReady (IBM) and AfterControlKeySent (Open Systems) events to show or hide a control when a specific screen is recognized. For more about handling events and using screen recognition to control macros, see Using Reflection Events and Controlling Macro Execution.
These samples show how to show or hide the control. Another option is to enable or disable ("gray out") the control.
'When the appropriate screen is ready, enable the control
If screenID = "INTERNATIONAL" Then
control.Enabled = True
Else
control.Enabled = False
End If
See Also